Android 알림 받는 기능
FCM(Firebase Cloud Messaging) Librar를 이용한 알림 기능입니다.
Android Studio Project 생성합니다.
Firebase 사이트 들어가 세팅작업하기
https://console.firebase.google.com/?pli=1
로그인 - Google 계정
하나의 계정으로 모든 Google 서비스를 Google 계정으로 로그인
accounts.google.com
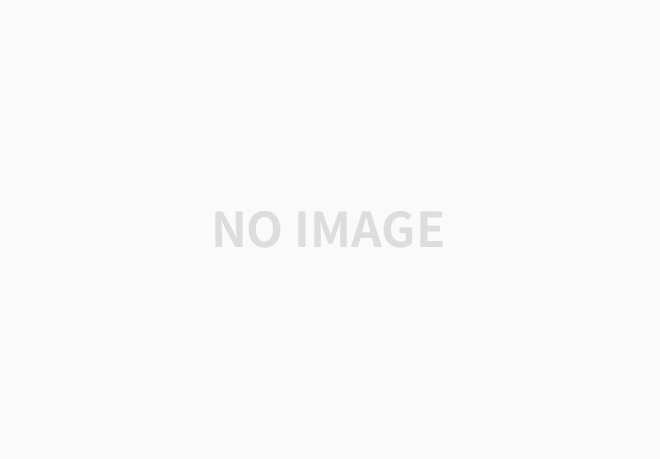
Firebase Project 생성하고 프로젝트에서 앱 추가를 하면 위에 이미지 처럼 나옵니다.
Android 패키지 이름은 Android Studio에 만든 패키지명과 동일하게 해야 합니다.
디버그 서명 인증서 SHA-1는 Android Studio 우측 Gradle 탭에 Tasks>android>signigReport 클릭하면 있습니다.
Firebase app 추가까지 하면 google-services.json 파일을 제공하는데 이 json 파일을 프로젝트 최상단에 넣어주시면 됩니다. (JSON 파일에 api 등 있음)
Android 기본 Setting은 Firebase Reference에 있습니다. (대충 적는다는 의미)
안드로이드 targetSdkVersion 31 (12)로 하면 뭔가 바껴서 에러남. PendingIntent 문제 발생!!
build.gradle : 프로젝트
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
jcenter()
}
dependencies {
classpath "com.android.tools.build:gradle:4.1.3"
classpath 'com.google.gms:google-services:4.3.10'
// NOTE: Do not place your application dependencies here; they belong
// in the individual module build.gradle files
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
build.gradle : 모듈
plugins {
id 'com.android.application'
id 'com.google.gms.google-services'
}
android {
compileSdkVersion 31
// buildToolsVersion "30.0.3"
buildToolsVersion "28.0.0"
defaultConfig {
applicationId "com.example.fcmandroid"
minSdkVersion 23
// targetSdkVersion 31
targetSdkVersion 28
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
implementation 'com.google.firebase:firebase-messaging:17.3.4'
implementation 'com.google.firebase:firebase-core:16.0.4'
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.fcmandroid">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.FCMAndroid">
<activity android:name=".MainActivity" android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<!-- app에 알림 받는 설정 -->
<service android:name=".MyFirebaseMessagingService" android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
</application>
</manifest>
MyFirbaseMessagingService
package com.example.fcmandroid;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Context;
import android.content.Intent;
import android.media.RingtoneManager;
import android.net.Uri;
import android.os.Build;
import android.os.Looper;
import android.util.Log;
import android.widget.Toast;
import androidx.annotation.NonNull;
import androidx.core.app.NotificationCompat;
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onNewToken(String s) {
super.onNewToken(s);
Log.e("Firebase", "FirebaseInstanceIDService : " + s);
}
/**
* 메시지 수신받는 메소드
* @param msg
*/
@Override
public void onMessageReceived(RemoteMessage msg) {
Log.d("### msg : ", msg.getNotification().getTitle());
if(msg.getData().isEmpty()){
sendNotification(msg.getNotification().getBody());
}else
sendNotification(msg.getData().get("content"));
}
private void sendNotification(String messageBody) {
Intent intent = new Intent(this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0 /* Request code */, intent,
PendingIntent.FLAG_ONE_SHOT);
String channelId = getString(R.string.default_notification_channel_id);
Uri defaultSoundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
NotificationCompat.Builder notificationBuilder =
new NotificationCompat.Builder(this, channelId)
.setSmallIcon(R.drawable.ic_stat_ic_notification)
.setContentTitle(getString(R.string.fcm_message))
.setContentText(messageBody)
.setAutoCancel(true)
.setSound(defaultSoundUri)
.setContentIntent(pendingIntent);
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
// Since android Oreo notification channel is needed.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(channelId,
"Channel human readable title",
NotificationManager.IMPORTANCE_DEFAULT);
notificationManager.createNotificationChannel(channel);
}
notificationManager.notify(0 /* ID of notification */, notificationBuilder.build());
}
}
onMessageReceived method가 Firebase Server에서 메세지가 요청 들어오면 작동하는 메소드이다.
GitHub - firebase/quickstart-android: Firebase Quickstart Samples for Android
Firebase Quickstart Samples for Android. Contribute to firebase/quickstart-android development by creating an account on GitHub.
github.com
알림 테스트는 Firebase 사이트에 참여에 Cloud Messaging에서 할 수 있습니다
테스트 메세지 전송을 위해 token 필요함